Python——设置索引
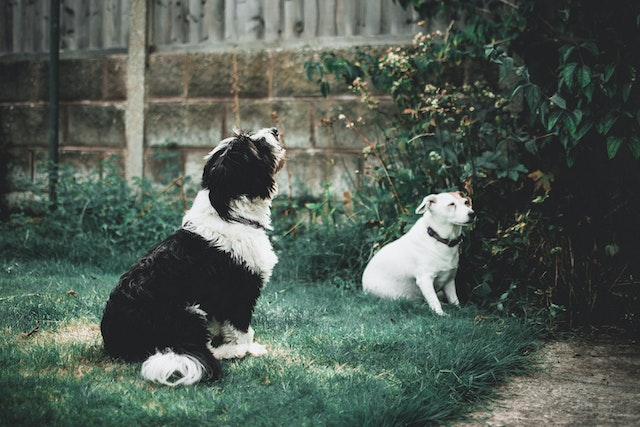
set_index( )
1 | DataFrame.set_index(keys, drop=True, append=False, inplace=False, verify_integrity=False) |
keys
:列标签或列标签/数组列表,需要设置为索引的列;
drop
:默认为True,删除用作新索引的列;
append
:是否将列附加到现有索引,默认为False;
inplace
:输入布尔值,表示当前操作是否对原数据生效,默认为False;
verify_integrity
:检查新索引的副本。否则,请将检查推迟到必要时进行。将其设置为false将提高该方法的性能,默认为false。
1 | import pandas as pd |
1 | name sex money class |
1、drop=True,删除用作新索引的列
2、append=False,将列附加到现有索引
1 | df_new = df.set_index('name',drop=True, append=False, inplace=False, verify_integrity=False) |
1 | sex money class |
1、drop=False,不删除用作新索引的列
2、append=False,将列附加到现有索引
1 | df_new1 = df.set_index('name',drop=False, append=False, inplace=False, verify_integrity=False) |
1 | name sex money class |
append=True,保留原索引和新索引
1 | df_new2 = df.set_index('name',drop=False, append=True, inplace=False, verify_integrity=False) |
1 | name sex money class |
reset_index()
1 | DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill='') |
evel
:数值类型可以为:int、str、tuple或list,默认无,仅从索引中删除给定级别。默认情况下移除所有级别。控制了具体要还原的那个等级的索引 。
drop
:当指定drop=False时,则索引列会被还原为普通列;否则,经设置后的新索引值被会丢弃。默认为False**。
inplace
:输入布尔值,表示当前操作是否对原数据生效,默认为False。
col_level
:数值类型为int或str,默认值为0,如果列有多个级别,则确定将标签插入到哪个级别。默认情况下,它将插入到第一级。
col_fill
:对象,默认‘’,如果列有多个级别,则确定其他级别的命名方式。如果没有,则重复索引名。注:eset_index()还原可分为两种类型,第一种是对原来的数据表进行reset;第二种是对使用过set_index()函数的数据表进行reset。
第一种方法
1 | df_new = df.set_index('name',drop=True, append=False, inplace=False, verify_integrity=False) |
1 | sex money class |
第二种方法
用reset_index()函数进行还原df_new
1 | df_new01 = df_new.reset_index(drop=False) |
1 | name sex money class |